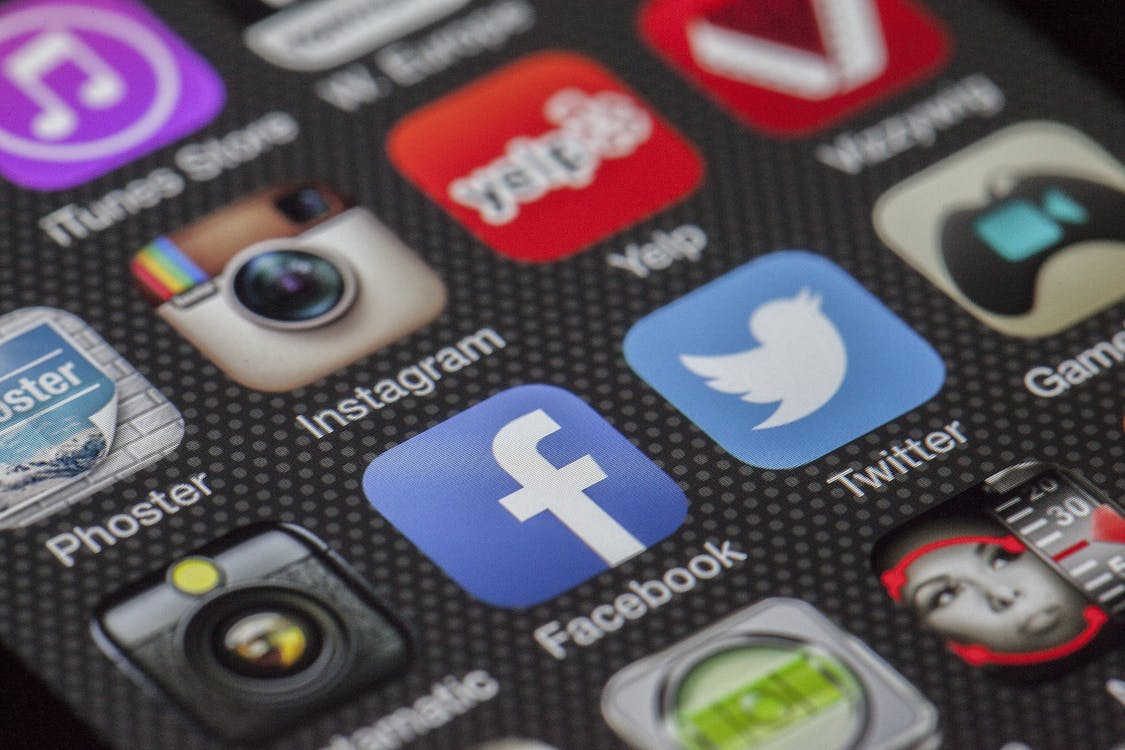
Boost Your Business with a Custom App
Boost your business with a custom mobile app from 4BIS Innovations. Increase sales, engage customers, and future-proof your success.
Search bars are a very common occurrence on websites. You see them everywhere in all different shapes and sizes. Some are just simple text search bars and some are large complex search bars that will query a database or make API calls.
In this article I will show you how to make a simple text search bar that searches a list of HTML elements.
First things first, create your HTML
<input type="text" placeholder="Search fruits..." id="searchBar">
<ul id="fruits-list">
<li>Apple</li>
<li>Pear</li>
<li>Banana</li>
<li>Orange</li>
</ul>
This code will print
a very simple list on the screen that will ook something like this:
Next, we can write a script tag below this code and start writing our JavaScript. We start with adding a “keyup” event listener to the text search bar. JavaScript allows you to write the same code in different ways. In this example I will use an “arrow function” and I get the search value from the “evt” parameter.
<script>
document.getElementById("searchbar").addEventlistener("keyup", evt => {
const searchValue = evt.target.value.toLowerCase()
})
</script>
Now every time you type inside the text field, the value of what you typed is parsed to lowercase letters and saved in the searchValue variable. The reason we use .toLowerCase() is to make the search bar non-case-sensitive.
Next thing we do; we select all the <li>
elements inside our
<ul>
element and loop over them to check their content.
<script>
document.getElementById("searchbar").addEventlistener("keyup", evt => {
const searchValue = evt.target.value.toLowerCase()
for (const li of document.querySelector("#fruits-list")) {
const content = li.innerText.toLowerCase()
li.style.display = content.includes(searchValue) ? "list-item" : "none"
}
})
</script>
The content of the
list item is it’s innerText, parsed to lowercase to ignore
case-sensitivity. To change the visibility of the <li>
element
we use a Conditional (ternary) operator. Conditional operators:
Operand one is a condition, the part after the “?” will
be used if condition is “truthy”, the part after “:” will be
used if the condition is ”falsy”.
This code will now show the element when it’s text contains your searched string, and hide it if when it does contain.
There is a lot of different ways to write this code and to achieve the same results. This article shows a very simple & compact JavaScript search bar.
Final code:
<input type="text" placeholder="Search fruits..." id="searchBar">
<ul id="fruits-list">
<li>Apple</li>
<li>Pear</li>
<li>Banana</li>
<li>Orange</li>
</ul>
<script>
document.getElementById("searchbar").addEventlistener("keyup", evt => {
const searchValue = evt.target.value.toLowerCase()
for (const li of document.querySelector("#fruits-list")) {
const content = li.innerText.toLowerCase()
li.style.display = content.includes(searchValue) ? "list-item" : "none"
}
})
</script>
Also interesting
Expand your knowledge with the 4BIS Blog...
Boost your business with a custom mobile app from 4BIS Innovations. Increase sales, engage customers, and future-proof your success.
Do you want to start a successful webshop? Find out all about strategy, technology, marketing and security. 4BIS Innovations helps you with custom solutions!
Discover high-quality, custom web development solutions at 4BIS Innovations in Maastricht. We specialize in building fast, secure, and user-friendly websites and web applications...
Do you want to know more or are you interested? Please feel free to contact us directly, by phone or via mail.
Or use one of our contact forms so that we can answer you as quickly and appropriately as possible can give. We would love to hear from you!